文章可能有更新,如需了解,请查看原文:从此不再担心键盘遮住输入框OC(一)
想必大家在iOS开发中都有遇到过这种问题。点击输入框后,弹出的键盘遮挡了输入框,然后你就无法看见你输入了什么。为了解决这个问题,我也在 Github、CocoaChina以及Code4App上花了不少时间去找相关的代码以及实现。
先来一张效果图
如何使用、源码分析
下面我通过如何使用
和源码分析
两个方面来介绍KeyboardToolBar。
如何使用
Podfile
platform :ios, '7.0'
pod 'KeyboardToolBar', '~> 1.0.0'
import
/// 不要忘了先导入.h
#import "KeyboardToolBar.h"
/// 使用该方法给UITextField注册使用KeyboardToolBar
/// @param textField 需要注册的UITextField
[KeyboardToolBar registerKeyboardToolBar:self.textField];
/// 不想让UITextField使用KeyboardToolBar的时候
/// 强烈建议使用该方法给UITextField移除KeyboardToolBar
/// @param textField 需要移除的UITextField
[KeyboardToolBar unregisterKeyboardToolBar:self.textField];
/// 如果嫌一个一个给UITextField移除KeyboardToolBar麻烦
/// 使用这个方法一次性将所有的UITextField移除KeyboardToolBar
[KeyboardToolBar unregisterAllKeyboardToolBar];
源码分析
实现思路
我的设想是在键盘上方的工具栏处做文章,哪怕是键盘挡住了输入框,但是如果将输入框上的placeholder
以及输入的内容时刻在工具栏上显示,那么哪怕键盘挡住了输入框,依旧可以清楚知道我现在要输入哪方面的内容以及我现在输入的内容是什么。
源码分析
为了看起来清楚些,以下代码中我使用KTB
代表KeyboardToolBar单例对象
。
.h文件
/// 继承iOS自带的UIToolbar
@interface KeyboardToolBar : UIToolbar
宏
/// KeyboardToolBar宽度
#define KeyboardToolBarWidth [UIScreen mainScreen].bounds.size.width
/// KeyboardToolBar高度
#define KeyboardToolBarHeight 44
/// KeyboardToolBar上UIScrollView组件的宽度
#define KeyboardScrollViewWidth (KeyboardToolBarWidth-80)
属性
@property(nonatomic, strong)UIScrollView *scrollView;
@property(nonatomic, strong)UITextField *toolBarTextField;
/// 字典用于存放注册使用KeyboardToolBar的所有UITextField
@property(nonatomic, retain)NSMutableDictionary *allRegisterTextFields;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| /// KTBstatic KeyboardToolBar *keyboardToolBar = nil; + (instancetype)shareKeyboardToolBar { if (keyboardToolBar == nil) { /// KTB是否初始化,如果没有,则进行初始化 /// KeyboardToolBar上需要有一个UIScrollView组件,UIScrollView内部有一个UITextField,如果UITextField内的内容过多,UIScrollView就派上用场了。 /// KeyboardToolBar的右侧还需要有一个'完成'按钮,点击该按钮后关闭键盘 keyboardToolBar = [[KeyboardToolBar alloc]initWithFrame:CGRectMake(0, 0, KeyboardToolBarWidth, KeyboardToolBarHeight)]; [keyboardToolBar setBarStyle:UIBarStyleDefault]; keyboardToolBar.scrollView = [[UIScrollView alloc] initWithFrame:CGRectMake(0, 0, KeyboardScrollViewWidth, KeyboardToolBarHeight)]; keyboardToolBar.scrollView.backgroundColor = [UIColor clearColor]; keyboardToolBar.scrollView.contentSize = CGSizeMake(KeyboardScrollViewWidth, KeyboardToolBarHeight); keyboardToolBar.scrollView.bounces = NO; keyboardToolBar.toolBarTextField = [[UITextField alloc] initWithFrame:CGRectMake(0, 0, KeyboardScrollViewWidth, KeyboardToolBarHeight)]; keyboardToolBar.toolBarTextField.textAlignment = NSTextAlignmentLeft; keyboardToolBar.toolBarTextField.userInteractionEnabled = NO; [keyboardToolBar.scrollView addSubview:keyboardToolBar.toolBarTextField]; UIBarButtonItem *textFieldItem = [[UIBarButtonItem alloc] initWithCustomView:keyboardToolBar.scrollView]; UIBarButtonItem *finishBtnItem = [[UIBarButtonItem alloc]initWithTitle:@"完成" style:UIBarButtonItemStyleDone target:keyboardToolBar action:@selector(resignKeyboard)]; NSArray * buttonsArray = [NSArray arrayWithObjects:textFieldItem,finishBtnItem,nil]; [keyboardToolBar setItems:buttonsArray]; } return keyboardToolBar; } /// 关闭键盘 - (void)resignKeyboard { keyboardToolBar.toolBarTextField.text = @""; [[[UIApplication sharedApplication] keyWindow] endEditing:YES]; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| + (void)registerKeyboardToolBar:(UITextField *)textField { if([KeyboardToolBar shareKeyboardToolBar].allRegisterTextFields == nil) { keyboardToolBar.allRegisterTextFields = [NSMutableDictionary dictionaryWithCapacity:10]; } /// 将KTB赋予传入的textField [textField setInputAccessoryView:keyboardToolBar]; /// 为传入的textField对象addTarget [textField addTarget:keyboardToolBar action:@selector(textFieldDidBegin:) forControlEvents:UIControlEventEditingDidBegin]; [textField addTarget:keyboardToolBar action:@selector(textFieldDidChange:) forControlEvents:UIControlEventEditingChanged]; /// 将传入的textField保存于KTB [keyboardToolBar.allRegisterTextFields setValue:textField forKey:[NSString stringWithFormat:@"%p",textField]]; } - (void)textFieldDidBegin:(UITextField *)textField { [self reSetTextField:textField]; } - (void)textFieldDidChange:(UITextField *)textField { [self reSetTextField:textField]; } /// 将textField的placeholder以及textField上的文字及时显示在KTB内部的UITextField上 - (void)reSetTextField:(UITextField *)textField { UITextField *tempTextField = [keyboardToolBar.allRegisterTextFields objectForKey:[NSString stringWithFormat:@"%p",textField]]; CGFloat textWidth = [KeyboardToolBar widthForString:tempTextField.text withFont:keyboardToolBar.toolBarTextField.font]; if(textWidth > KeyboardScrollViewWidth) { keyboardToolBar.toolBarTextField.frame = CGRectMake(0,0,textWidth,KeyboardToolBarHeight); keyboardToolBar.scrollView.contentSize = CGSizeMake(textWidth, KeyboardToolBarHeight); [self.scrollView scrollRectToVisible:CGRectMake(textWidth-KeyboardScrollViewWidth,0,KeyboardScrollViewWidth,KeyboardToolBarHeight) animated:YES]; } else { keyboardToolBar.toolBarTextField.frame = CGRectMake(0, 0, KeyboardScrollViewWidth, KeyboardToolBarHeight); keyboardToolBar.scrollView.contentSize = CGSizeMake(KeyboardScrollViewWidth, KeyboardToolBarHeight); } keyboardToolBar.toolBarTextField.text = tempTextField.text; keyboardToolBar.toolBarTextField.textColor = tempTextField.textColor; if(tempTextField.placeholder != nil) { NSAttributedString *attribute = textField.attributedPlaceholder; NSDictionary *dictionary = [attribute attributesAtIndex:0 effectiveRange:nil]; keyboardToolBar.toolBarTextField.attributedPlaceholder = [[NSAttributedString alloc] initWithString:tempTextField.placeholder attributes:@{NSForegroundColorAttributeName: [dictionary valueForKey:NSForegroundColorAttributeName]}]; } } /// 根据文本内容和字体计算NSString长度用于设置KTB内部的UIScrollView以及UITextField的宽度 + (CGFloat)widthForString:(NSString *)str withFont:(UIFont *)font { NSDictionary *attribute = @{NSFontAttributeName: font}; CGSize size = [str boundingRectWithSize:CGSizeMake(CGFLOAT_MAX, CGFLOAT_MAX) options: NSStringDrawingTruncatesLastVisibleLine | NSStringDrawingUsesLineFragmentOrigin | NSStringDrawingUsesFontLeading attributes:attribute context:nil].size; return size.width; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| /// 直接将KTB中所有已注册的UITextField移除 + (void)unregisterKeyboardToolBar:(UITextField *)textField { if(keyboardToolBar == nil || keyboardToolBar.allRegisterTextFields.count == 0) { return; } UITextField *tempTextField = [keyboardToolBar.allRegisterTextFields objectForKey:[NSString stringWithFormat:@"%p",textField]]; [tempTextField setInputAccessoryView:nil]; [tempTextField removeTarget:keyboardToolBar action:@selector(textFieldDidBegin:) forControlEvents:UIControlEventEditingDidBegin]; [tempTextField removeTarget:keyboardToolBar action:@selector(textFieldDidChange:) forControlEvents:UIControlEventEditingChanged]; [keyboardToolBar.allRegisterTextFields removeObjectForKey:[NSString stringWithFormat:@"%p",textField]]; if(keyboardToolBar.allRegisterTextFields.count == 0) { keyboardToolBar.allRegisterTextFields = nil; keyboardToolBar = nil; } } /// 根据传入的UITextField兑现。从KTB内移除 + (void)unregisterAllKeyboardToolBar { if(keyboardToolBar == nil || keyboardToolBar.allRegisterTextFields.count == 0) { return; } NSEnumerator *enumeratorValue = [keyboardToolBar.allRegisterTextFields objectEnumerator]; for(UITextField *tempTextField in enumeratorValue) { [tempTextField setInputAccessoryView:nil]; [tempTextField removeTarget:keyboardToolBar action:@selector(textFieldDidBegin:) forControlEvents:UIControlEventEditingDidBegin]; [tempTextField removeTarget:keyboardToolBar action:@selector(textFieldDidChange:) forControlEvents:UIControlEventEditingChanged]; } [keyboardToolBar.allRegisterTextFields removeAllObjects]; keyboardToolBar.allRegisterTextFields = nil; keyboardToolBar = nil; }
|
好了,以上就是我的介绍,欢迎大家来我的KeyboardToolBar主页进行Star、Issues或Pull requests,我是Jiar,我热爱交朋友~
微信订阅号
欢迎关注我的个人微信订阅号,我将不定期分享开发方面的干货。
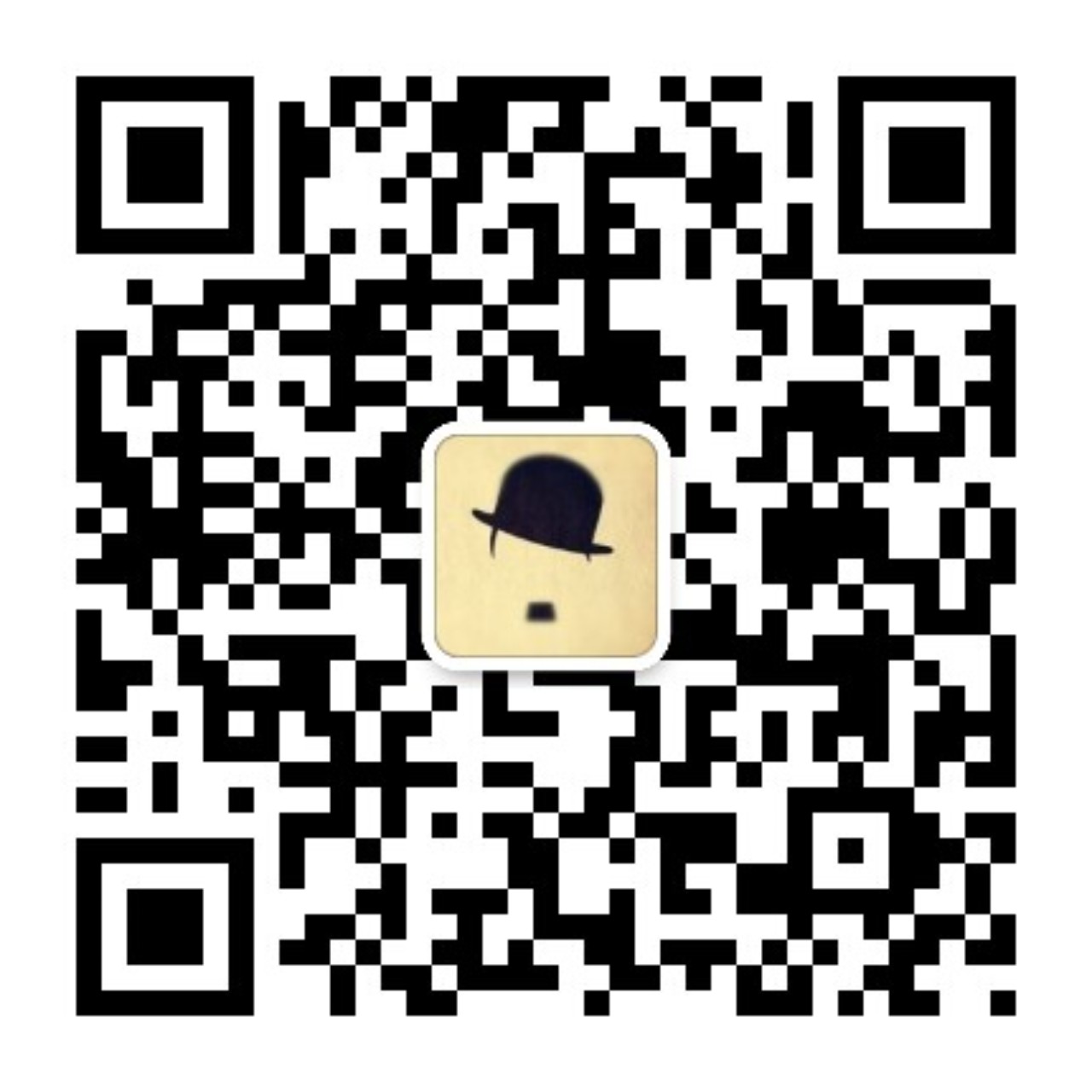